Note
Go to the end to download the full example code.
Apple Picker¶
We demonstrate ASPIRE’s particle picking methods using the Apple
class.
import os
import matplotlib.pyplot as plt
import mrcfile
from aspire.apple.apple import Apple
Read and Plot Micrograph¶
Here we demonstrate reading in and plotting a raw micrograph.
file_path = os.path.join(
os.path.dirname(os.getcwd()), "data", "falcon_2012_06_12-14_33_35_0.mrc"
)
with mrcfile.open(file_path, mode="r") as mrc:
micro_img = mrc.data
plt.title("Sample Micrograph")
plt.imshow(micro_img, cmap="gray")
plt.show()
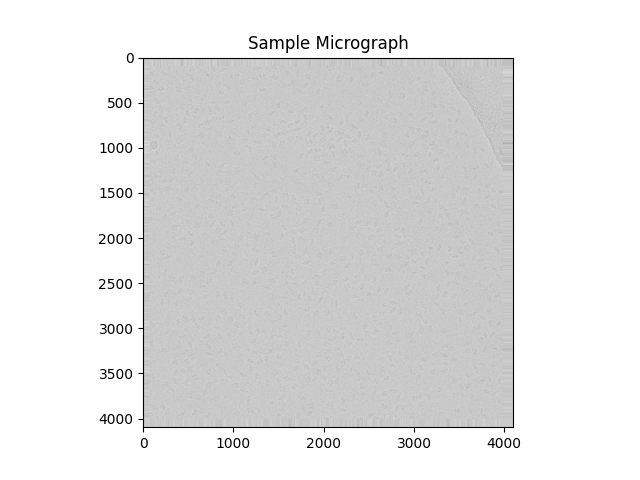
Initialize Apple¶
Initiate ASPIRE’s Apple
class.
Apple
admits many options relating to particle sizing and mrc processing.
apple_picker = Apple(
particle_size=78, min_particle_size=19, max_particle_size=156, tau1=710, tau2=7100
)
Pick Particles and Find Centers¶
Here we use the process_micrograph
method from the Apple
class to find particles in the micrograph.
It will also return an image suitable for display, and optionally save a jpg.
centers, particles_img = apple_picker.process_micrograph(file_path, create_jpg=True)
# Note that if you only desire ``centers`` you may call ``process_micrograph_centers(file_path,...)``.
0%| | 0/256 [00:00<?, ?it/s]
0%| | 1/256 [00:00<01:41, 2.50it/s]
2%|▏ | 4/256 [00:00<00:30, 8.24it/s]
2%|▏ | 6/256 [00:00<00:33, 7.36it/s]
3%|▎ | 8/256 [00:01<00:30, 8.06it/s]
4%|▎ | 9/256 [00:01<00:33, 7.34it/s]
4%|▍ | 10/256 [00:01<00:32, 7.66it/s]
4%|▍ | 11/256 [00:01<00:34, 7.05it/s]
5%|▌ | 13/256 [00:01<00:31, 7.68it/s]
6%|▌ | 15/256 [00:01<00:28, 8.39it/s]
7%|▋ | 17/256 [00:02<00:28, 8.39it/s]
7%|▋ | 19/256 [00:02<00:32, 7.38it/s]
8%|▊ | 21/256 [00:02<00:25, 9.22it/s]
9%|▉ | 23/256 [00:03<00:31, 7.36it/s]
10%|▉ | 25/256 [00:03<00:25, 9.01it/s]
11%|█ | 27/256 [00:03<00:29, 7.83it/s]
11%|█▏ | 29/256 [00:03<00:26, 8.41it/s]
12%|█▏ | 31/256 [00:03<00:27, 8.31it/s]
13%|█▎ | 33/256 [00:04<00:30, 7.29it/s]
14%|█▍ | 36/256 [00:04<00:25, 8.65it/s]
14%|█▍ | 37/256 [00:04<00:29, 7.42it/s]
15%|█▌ | 39/256 [00:04<00:23, 9.09it/s]
16%|█▌ | 41/256 [00:05<00:25, 8.49it/s]
16%|█▋ | 42/256 [00:05<00:26, 8.03it/s]
17%|█▋ | 44/256 [00:05<00:26, 7.89it/s]
18%|█▊ | 46/256 [00:05<00:26, 7.89it/s]
19%|█▉ | 48/256 [00:06<00:26, 7.80it/s]
20%|█▉ | 50/256 [00:06<00:25, 8.17it/s]
20%|██ | 52/256 [00:06<00:23, 8.54it/s]
21%|██ | 54/256 [00:06<00:27, 7.38it/s]
22%|██▏ | 57/256 [00:07<00:22, 8.72it/s]
23%|██▎ | 58/256 [00:07<00:25, 7.65it/s]
23%|██▎ | 60/256 [00:07<00:22, 8.83it/s]
24%|██▍ | 61/256 [00:07<00:26, 7.30it/s]
25%|██▍ | 63/256 [00:07<00:20, 9.29it/s]
25%|██▌ | 65/256 [00:08<00:25, 7.49it/s]
26%|██▌ | 67/256 [00:08<00:21, 8.86it/s]
27%|██▋ | 69/256 [00:08<00:20, 8.93it/s]
28%|██▊ | 71/256 [00:08<00:22, 8.23it/s]
28%|██▊ | 72/256 [00:08<00:22, 8.11it/s]
29%|██▉ | 74/256 [00:09<00:25, 7.15it/s]
30%|███ | 77/256 [00:09<00:20, 8.57it/s]
30%|███ | 78/256 [00:09<00:24, 7.38it/s]
32%|███▏ | 81/256 [00:10<00:21, 8.06it/s]
32%|███▏ | 83/256 [00:10<00:20, 8.48it/s]
33%|███▎ | 84/256 [00:10<00:19, 8.63it/s]
33%|███▎ | 85/256 [00:10<00:23, 7.24it/s]
34%|███▍ | 87/256 [00:10<00:18, 9.17it/s]
35%|███▍ | 89/256 [00:11<00:20, 8.28it/s]
35%|███▌ | 90/256 [00:11<00:21, 7.72it/s]
36%|███▌ | 92/256 [00:11<00:20, 7.81it/s]
36%|███▋ | 93/256 [00:11<00:20, 8.15it/s]
37%|███▋ | 95/256 [00:11<00:18, 8.93it/s]
38%|███▊ | 96/256 [00:12<00:23, 6.83it/s]
39%|███▊ | 99/256 [00:12<00:19, 7.93it/s]
39%|███▉ | 100/256 [00:12<00:20, 7.73it/s]
40%|███▉ | 102/256 [00:12<00:16, 9.23it/s]
40%|████ | 103/256 [00:12<00:19, 8.00it/s]
41%|████ | 105/256 [00:13<00:18, 8.26it/s]
42%|████▏ | 107/256 [00:13<00:18, 8.13it/s]
43%|████▎ | 109/256 [00:13<00:18, 8.13it/s]
43%|████▎ | 110/256 [00:13<00:17, 8.20it/s]
43%|████▎ | 111/256 [00:13<00:18, 8.03it/s]
44%|████▍ | 113/256 [00:14<00:17, 8.17it/s]
45%|████▍ | 115/256 [00:14<00:17, 8.19it/s]
46%|████▌ | 117/256 [00:14<00:16, 8.40it/s]
46%|████▋ | 119/256 [00:14<00:17, 7.95it/s]
47%|████▋ | 121/256 [00:15<00:16, 7.95it/s]
48%|████▊ | 123/256 [00:15<00:16, 8.24it/s]
49%|████▉ | 125/256 [00:15<00:16, 7.86it/s]
50%|████▉ | 127/256 [00:15<00:15, 8.27it/s]
50%|█████ | 128/256 [00:15<00:15, 8.38it/s]
50%|█████ | 129/256 [00:16<00:16, 7.66it/s]
51%|█████ | 131/256 [00:16<00:16, 7.80it/s]
52%|█████▏ | 133/256 [00:16<00:15, 8.12it/s]
53%|█████▎ | 135/256 [00:16<00:15, 7.95it/s]
54%|█████▎ | 137/256 [00:17<00:14, 7.94it/s]
54%|█████▍ | 138/256 [00:17<00:14, 8.14it/s]
54%|█████▍ | 139/256 [00:17<00:14, 8.19it/s]
55%|█████▌ | 141/256 [00:17<00:14, 8.12it/s]
56%|█████▌ | 143/256 [00:17<00:14, 7.94it/s]
57%|█████▋ | 145/256 [00:18<00:13, 8.36it/s]
57%|█████▋ | 147/256 [00:18<00:14, 7.47it/s]
59%|█████▊ | 150/256 [00:18<00:12, 8.76it/s]
59%|█████▉ | 151/256 [00:18<00:13, 7.57it/s]
60%|█████▉ | 153/256 [00:18<00:11, 9.13it/s]
61%|██████ | 155/256 [00:19<00:12, 8.10it/s]
61%|██████▏ | 157/256 [00:19<00:12, 7.78it/s]
62%|██████▏ | 158/256 [00:19<00:12, 7.95it/s]
62%|██████▏ | 159/256 [00:19<00:11, 8.29it/s]
63%|██████▎ | 161/256 [00:20<00:11, 7.93it/s]
64%|██████▎ | 163/256 [00:20<00:11, 8.22it/s]
64%|██████▍ | 165/256 [00:20<00:11, 7.76it/s]
65%|██████▌ | 167/256 [00:20<00:11, 7.95it/s]
66%|██████▌ | 168/256 [00:20<00:11, 7.52it/s]
66%|██████▋ | 170/256 [00:21<00:09, 9.40it/s]
67%|██████▋ | 172/256 [00:21<00:09, 8.79it/s]
68%|██████▊ | 173/256 [00:21<00:10, 7.75it/s]
68%|██████▊ | 175/256 [00:21<00:10, 7.56it/s]
69%|██████▉ | 177/256 [00:21<00:09, 8.13it/s]
70%|██████▉ | 179/256 [00:22<00:09, 8.23it/s]
71%|███████ | 181/256 [00:22<00:09, 8.22it/s]
71%|███████▏ | 183/256 [00:22<00:10, 7.07it/s]
73%|███████▎ | 186/256 [00:23<00:08, 8.72it/s]
73%|███████▎ | 187/256 [00:23<00:08, 7.75it/s]
74%|███████▍ | 189/256 [00:23<00:07, 9.55it/s]
75%|███████▍ | 191/256 [00:23<00:08, 7.87it/s]
75%|███████▌ | 193/256 [00:23<00:07, 8.40it/s]
76%|███████▌ | 194/256 [00:24<00:08, 7.54it/s]
77%|███████▋ | 196/256 [00:24<00:06, 8.68it/s]
77%|███████▋ | 197/256 [00:24<00:08, 7.29it/s]
78%|███████▊ | 199/256 [00:24<00:06, 9.35it/s]
79%|███████▊ | 201/256 [00:24<00:06, 7.94it/s]
79%|███████▉ | 203/256 [00:25<00:06, 8.47it/s]
80%|███████▉ | 204/256 [00:25<00:07, 7.39it/s]
81%|████████ | 207/256 [00:25<00:06, 7.44it/s]
82%|████████▏ | 209/256 [00:25<00:05, 9.16it/s]
82%|████████▏ | 211/256 [00:26<00:05, 7.57it/s]
84%|████████▎ | 214/256 [00:26<00:05, 7.71it/s]
84%|████████▍ | 216/256 [00:26<00:04, 9.10it/s]
85%|████████▌ | 218/256 [00:26<00:04, 8.84it/s]
86%|████████▌ | 220/256 [00:27<00:04, 7.37it/s]
87%|████████▋ | 222/256 [00:27<00:03, 8.75it/s]
88%|████████▊ | 224/256 [00:27<00:04, 7.63it/s]
88%|████████▊ | 226/256 [00:27<00:03, 8.49it/s]
89%|████████▉ | 228/256 [00:28<00:03, 7.76it/s]
90%|████████▉ | 230/256 [00:28<00:03, 8.26it/s]
91%|█████████ | 232/256 [00:28<00:03, 7.29it/s]
92%|█████████▏| 235/256 [00:29<00:02, 8.74it/s]
92%|█████████▏| 236/256 [00:29<00:02, 7.90it/s]
93%|█████████▎| 238/256 [00:29<00:02, 8.52it/s]
94%|█████████▍| 240/256 [00:29<00:02, 7.32it/s]
95%|█████████▍| 243/256 [00:30<00:01, 8.66it/s]
95%|█████████▌| 244/256 [00:30<00:01, 7.64it/s]
96%|█████████▌| 246/256 [00:30<00:01, 9.02it/s]
96%|█████████▋| 247/256 [00:30<00:01, 7.68it/s]
97%|█████████▋| 249/256 [00:30<00:00, 8.74it/s]
98%|█████████▊| 250/256 [00:30<00:00, 8.85it/s]
98%|█████████▊| 251/256 [00:31<00:00, 7.82it/s]
99%|█████████▉| 253/256 [00:31<00:00, 8.11it/s]
99%|█████████▉| 254/256 [00:31<00:00, 8.43it/s]
100%|█████████▉| 255/256 [00:31<00:00, 8.42it/s]
100%|██████████| 256/256 [00:31<00:00, 8.11it/s]
Plot the Picked Particles¶
Observe the number of particles picked and plot the result from Apple
.
img_dim = micro_img.shape
particles = centers.shape[0]
print(f"Dimensions of the micrograph are {img_dim}")
print(f"{particles} particles were picked")
plt.imshow(particles_img, cmap="gray")
plt.show()
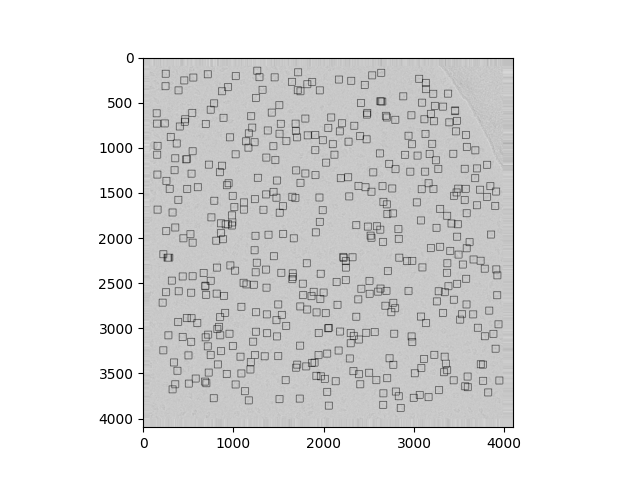
Dimensions of the micrograph are (4096, 4096)
461 particles were picked
Total running time of the script: (0 minutes 43.962 seconds)