Note
Go to the end to download the full example code.
Ab-initio Pipeline Demonstration¶
This tutorial demonstrates some key components of an ab-initio
reconstruction pipeline using synthetic data generated with ASPIRE’s
Simulation
class of objects.
Download an Example Volume¶
We begin by downloading a high resolution volume map of the 80S Ribosome, sourced from EMDB: https://www.ebi.ac.uk/emdb/EMD-2660. This is one of several volume maps that can be downloaded with ASPIRE’s data downloading utility by using the following import. sphinx_gallery_start_ignore flake8: noqa sphinx_gallery_end_ignore
from aspire.downloader import emdb_2660
# Load 80s Ribosome as a ``Volume`` object.
original_vol = emdb_2660()
# Downsample the volume
res = 41
vol = original_vol.downsample(res)
Note
A Volume
can be saved using the Volume.save()
method as follows:
fn = f"downsampled_80s_ribosome_size{res}.mrc"
vol.save(fn, overwrite=True)
Create a Simulation Source¶
ASPIRE’s Simulation
class can be used to generate a synthetic
dataset of projection images. A Simulation
object produces
random projections of a supplied Volume and applies noise and CTF
filters. The resulting stack of 2D images is stored in an Image
object.
CTF Filters¶
Let’s start by creating CTF filters. The operators
package
contains a collection of filter classes that can be supplied to a
Simulation
. We use RadialCTFFilter
to generate a set of CTF
filters with various defocus values.
# Create CTF filters
import numpy as np
from aspire.operators import RadialCTFFilter
# Radial CTF Filter
defocus_min = 15000 # unit is angstroms
defocus_max = 25000
defocus_ct = 7
ctf_filters = [
RadialCTFFilter(pixel_size=vol.pixel_size, defocus=d)
for d in np.linspace(defocus_min, defocus_max, defocus_ct)
]
Initialize Simulation Object¶
We feed our Volume
and filters into Simulation
to generate
the dataset of images. When controlled white Gaussian noise is
desired, WhiteNoiseAdder.from_snr()
can be used to generate a
simulation data set around a specific SNR.
Alternatively, users can bring their own images using an
ArrayImageSource
, or define their own custom noise functions via
Simulation(..., noise_adder=CustomNoiseAdder(...))
. Examples
can be found in tutorials/class_averaging.py
and
experiments/simulated_abinitio_pipeline.py
.
from aspire.noise import WhiteNoiseAdder
from aspire.source import Simulation
# set parameters
n_imgs = 2500
# SNR target for white gaussian noise.
snr = 0.5
Note
Note, the SNR value was chosen based on other parameters for this quick tutorial, and can be changed to adjust the power of the additive noise.
# For this ``Simulation`` we set all 2D offset vectors to zero,
# but by default offset vectors will be randomly distributed.
src = Simulation(
n=n_imgs, # number of projections
vols=vol, # volume source
offsets=0, # Default: images are randomly shifted
unique_filters=ctf_filters,
noise_adder=WhiteNoiseAdder.from_snr(snr=snr), # desired SNR
)
0%| | 0/5 [00:00<?, ?it/s]
20%|██ | 1/5 [00:00<00:00, 4.54it/s]
40%|████ | 2/5 [00:00<00:00, 4.55it/s]
60%|██████ | 3/5 [00:00<00:00, 4.63it/s]
80%|████████ | 4/5 [00:00<00:00, 4.64it/s]
100%|██████████| 5/5 [00:01<00:00, 4.83it/s]
100%|██████████| 5/5 [00:01<00:00, 4.73it/s]
Several Views of the Projection Images¶
We can access several views of the projection images.
# with no corruption applied
src.projections[0:10].show()
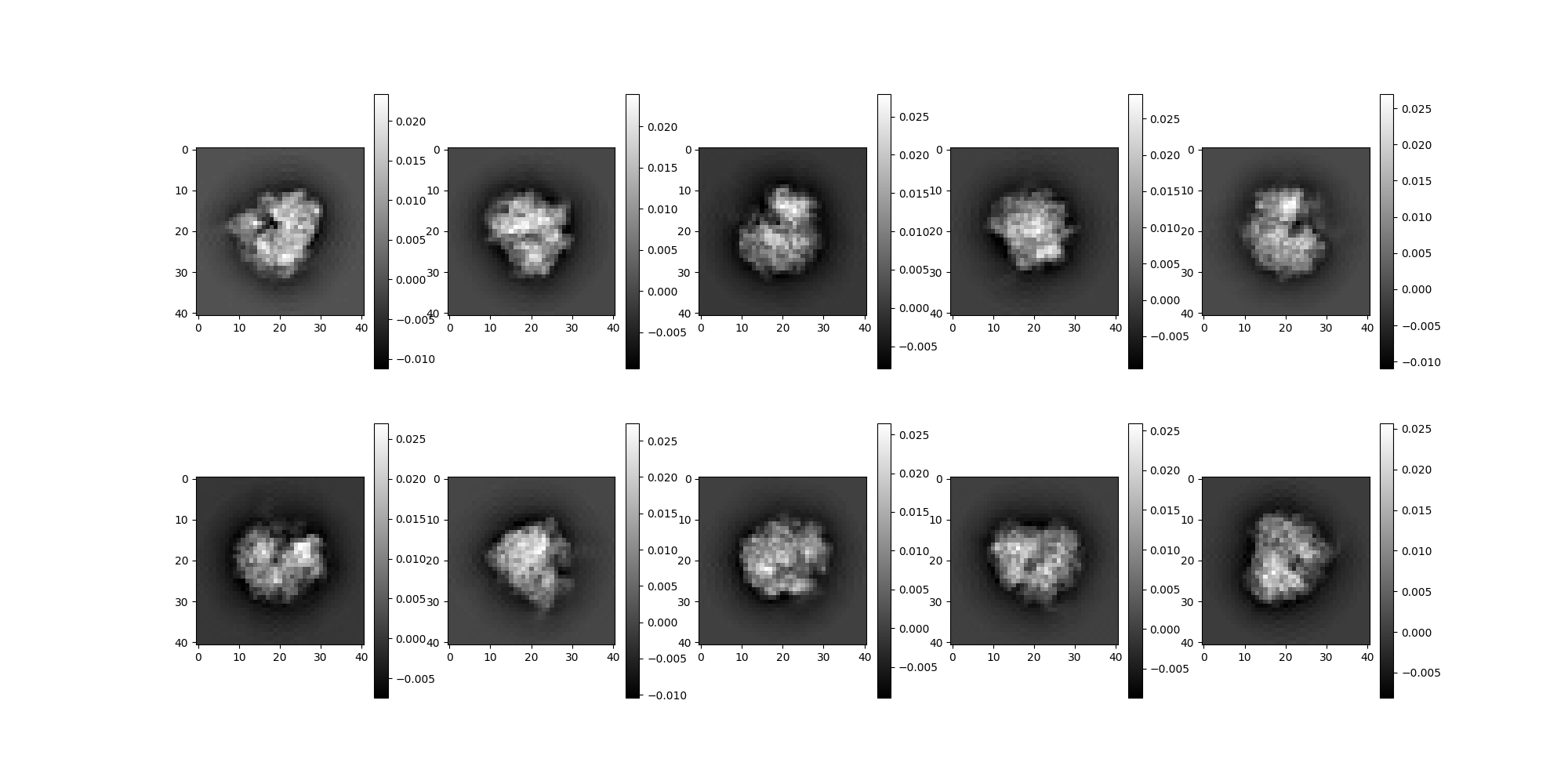
# with no noise corruption
src.clean_images[0:10].show()
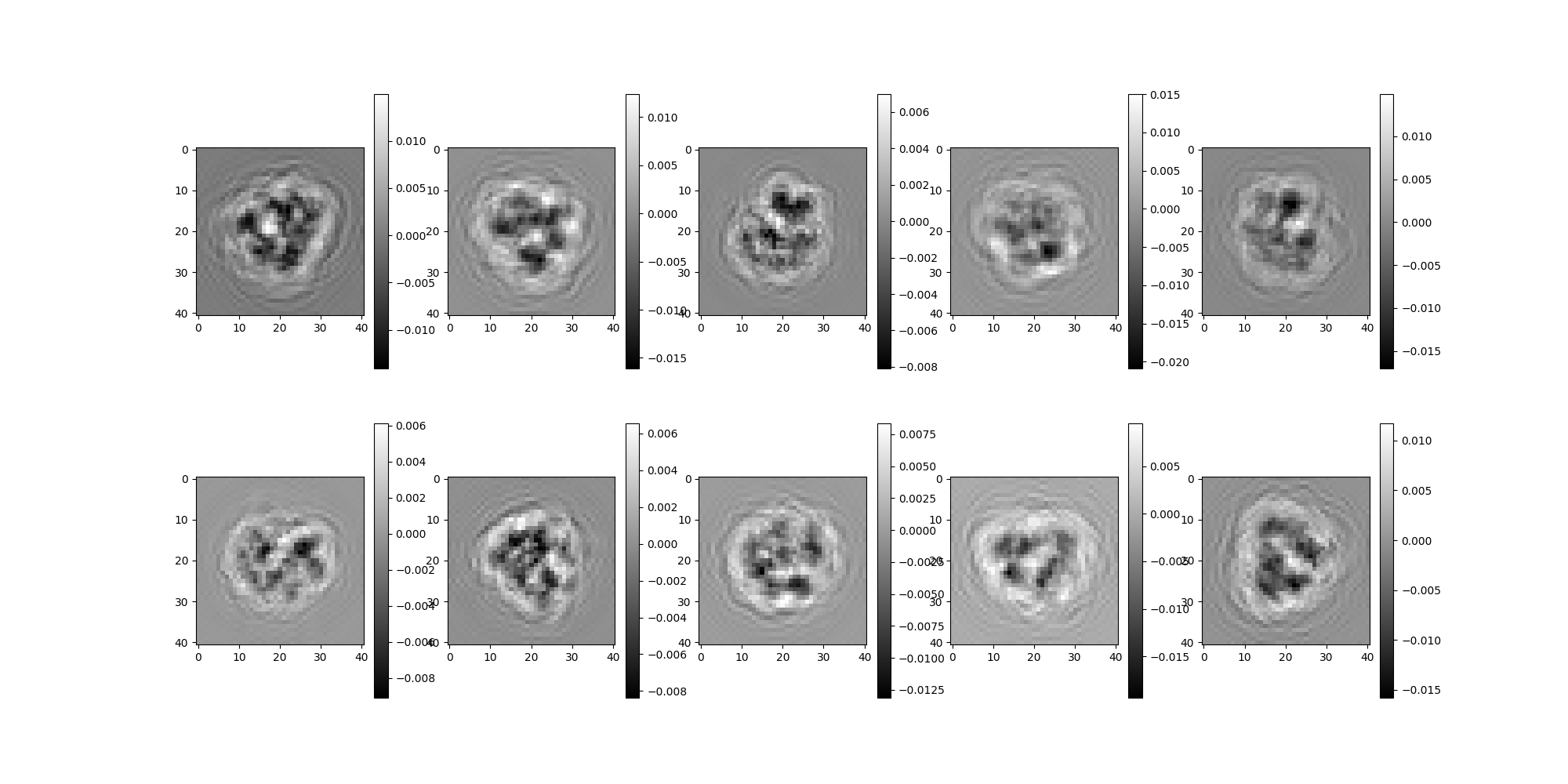
# with noise and CTF corruption
src.images[0:10].show()
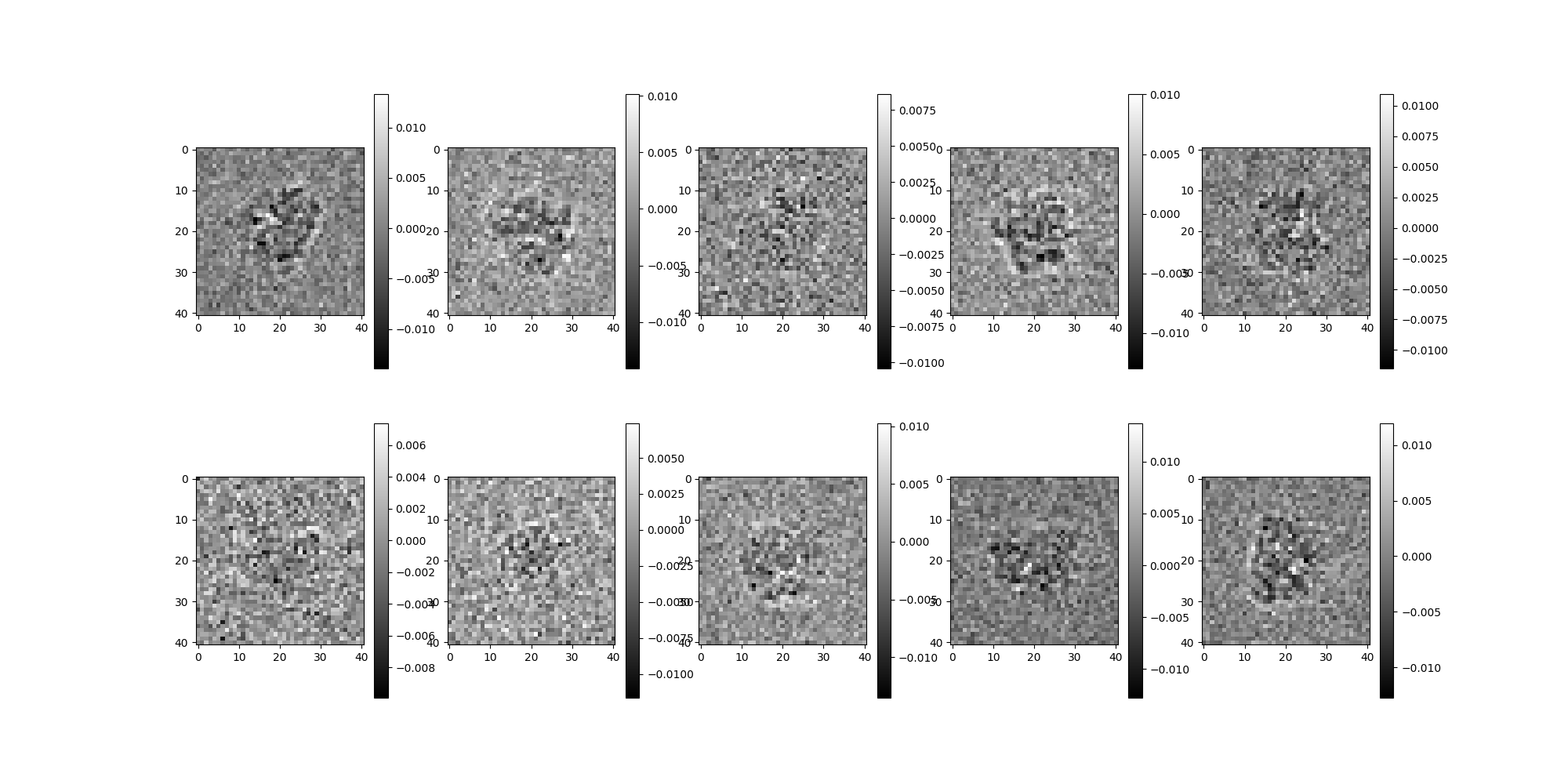
CTF Correction¶
We apply phase_flip()
to correct for CTF effects.
src = src.phase_flip()
Cache¶
We apply cache
to store the results of the ImageSource
pipeline up to this point. This is optional, but can provide
benefit when used intently on machines with adequate memory.
src = src.cache()
src.images[0:10].show()
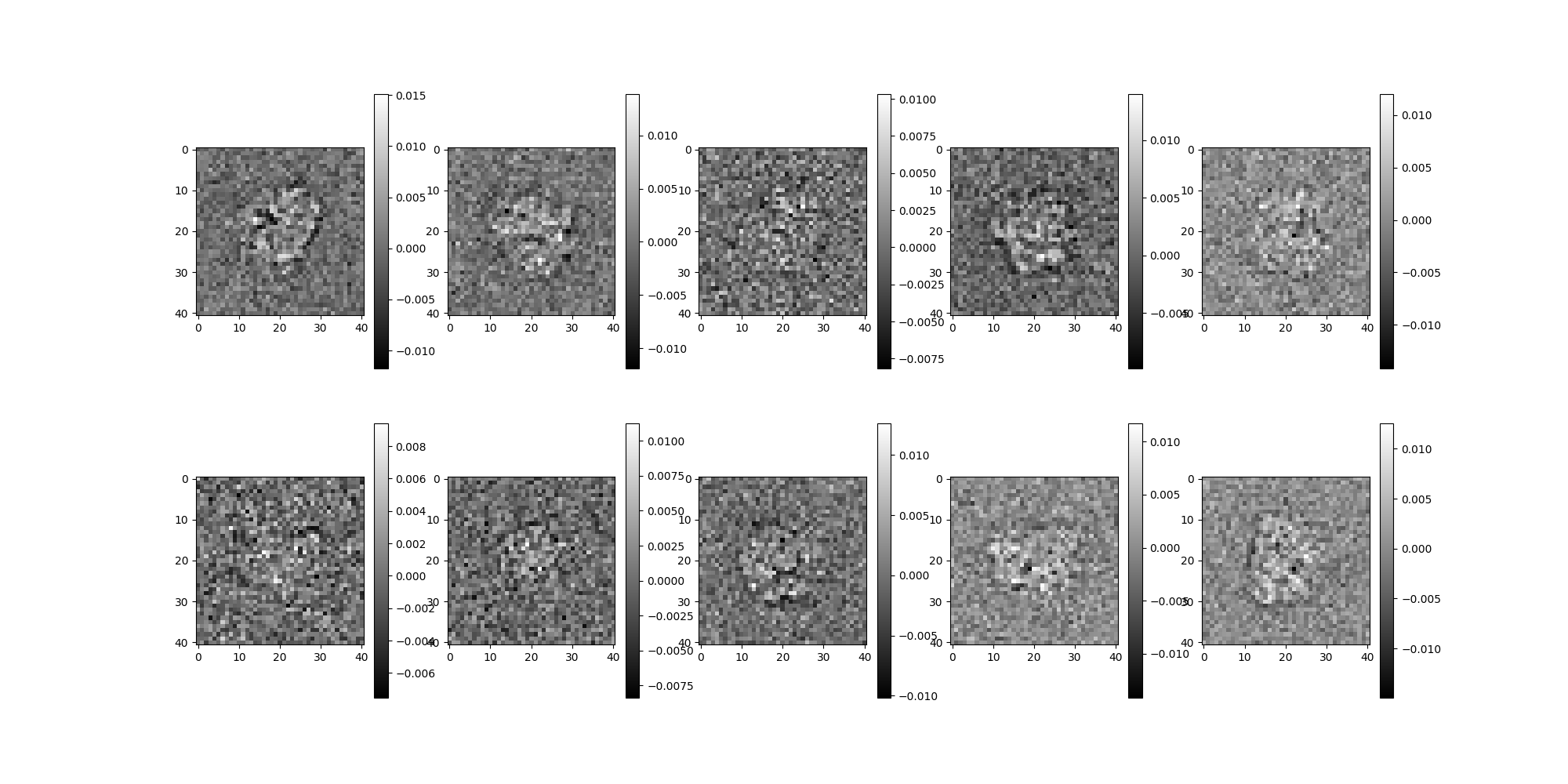
0%| | 0/5 [00:00<?, ?it/s]
20%|██ | 1/5 [00:00<00:03, 1.09it/s]
40%|████ | 2/5 [00:01<00:02, 1.09it/s]
60%|██████ | 3/5 [00:02<00:01, 1.10it/s]
80%|████████ | 4/5 [00:03<00:00, 1.10it/s]
100%|██████████| 5/5 [00:04<00:00, 1.15it/s]
100%|██████████| 5/5 [00:04<00:00, 1.12it/s]
Class Averaging¶
We use RIRClass2D
object to classify the images via the
rotationally invariant representation (RIR) algorithm. Class
selection is customizable. The classification module also includes a
set of protocols for selecting a set of images to be used after
classification. Here we’re using the simplest
DebugClassAvgSource
which internally uses the
TopClassSelector
to select the first n_classes
images from
the source. In practice, the selection is done by sorting class
averages based on some configurable notion of quality (contrast,
neighbor distance etc).
from aspire.classification import RIRClass2D
# set parameters
n_classes = 200
n_nbor = 6
# We will customize our class averaging source. Note that the
# ``fspca_components`` and ``bispectrum_components`` were selected for
# this small tutorial.
rir = RIRClass2D(
src,
fspca_components=40,
bispectrum_components=30,
n_nbor=n_nbor,
)
from aspire.denoising import DebugClassAvgSource
avgs = DebugClassAvgSource(
src=src,
classifier=rir,
)
# We'll continue our pipeline using only the first ``n_classes`` from
# ``avgs``. The ``cache()`` call is used here to precompute results
# for the ``:n_classes`` slice. This avoids recomputing the same
# images twice when peeking in the next cell then requesting them in
# the following ``CLSyncVoting`` algorithm. Outside of demonstration
# purposes, where we are repeatedly peeking at various stage results,
# such caching can be dropped allowing for more lazy evaluation.
avgs = avgs[:n_classes].cache()
0%| | 0/1 [00:00<?, ?it/s]
0%| | 0/5 [00:00<?, ?it/s]
100%|██████████| 5/5 [00:00<00:00, 700.26it/s]
0%| | 0/5 [00:00<?, ?it/s]
20%|██ | 1/5 [00:00<00:00, 6.04it/s]
40%|████ | 2/5 [00:00<00:00, 6.11it/s]
60%|██████ | 3/5 [00:00<00:00, 6.19it/s]
80%|████████ | 4/5 [00:00<00:00, 6.09it/s]
100%|██████████| 5/5 [00:00<00:00, 6.44it/s]
100%|██████████| 5/5 [00:00<00:00, 6.29it/s]
Rotationally aligning classes: 0%| | 0/200 [00:00<?, ?it/s]
Rotationally aligning classes: 2%|▏ | 3/200 [00:00<00:11, 17.24it/s]
Rotationally aligning classes: 2%|▎ | 5/200 [00:00<00:17, 11.19it/s]
Rotationally aligning classes: 4%|▎ | 7/200 [00:00<00:20, 9.64it/s]
Rotationally aligning classes: 4%|▍ | 9/200 [00:00<00:21, 9.09it/s]
Rotationally aligning classes: 5%|▌ | 10/200 [00:01<00:21, 8.88it/s]
Rotationally aligning classes: 6%|▌ | 11/200 [00:01<00:21, 8.74it/s]
Rotationally aligning classes: 6%|▋ | 13/200 [00:01<00:19, 9.41it/s]
Rotationally aligning classes: 7%|▋ | 14/200 [00:01<00:20, 9.10it/s]
Rotationally aligning classes: 8%|▊ | 15/200 [00:01<00:20, 8.90it/s]
Rotationally aligning classes: 8%|▊ | 17/200 [00:01<00:19, 9.46it/s]
Rotationally aligning classes: 9%|▉ | 18/200 [00:01<00:19, 9.13it/s]
Rotationally aligning classes: 10%|▉ | 19/200 [00:02<00:20, 8.92it/s]
Rotationally aligning classes: 10%|█ | 20/200 [00:02<00:20, 8.85it/s]
Rotationally aligning classes: 10%|█ | 21/200 [00:02<00:20, 8.79it/s]
Rotationally aligning classes: 11%|█ | 22/200 [00:02<00:20, 8.62it/s]
Rotationally aligning classes: 12%|█▏ | 23/200 [00:02<00:20, 8.51it/s]
Rotationally aligning classes: 12%|█▏ | 24/200 [00:02<00:20, 8.55it/s]
Rotationally aligning classes: 12%|█▎ | 25/200 [00:02<00:20, 8.48it/s]
Rotationally aligning classes: 13%|█▎ | 26/200 [00:02<00:20, 8.54it/s]
Rotationally aligning classes: 14%|█▎ | 27/200 [00:02<00:20, 8.50it/s]
Rotationally aligning classes: 14%|█▍ | 28/200 [00:03<00:20, 8.38it/s]
Rotationally aligning classes: 14%|█▍ | 29/200 [00:03<00:20, 8.28it/s]
Rotationally aligning classes: 15%|█▌ | 30/200 [00:03<00:20, 8.23it/s]
Rotationally aligning classes: 16%|█▌ | 31/200 [00:03<00:20, 8.26it/s]
Rotationally aligning classes: 16%|█▌ | 32/200 [00:03<00:20, 8.16it/s]
Rotationally aligning classes: 16%|█▋ | 33/200 [00:03<00:20, 8.15it/s]
Rotationally aligning classes: 17%|█▋ | 34/200 [00:03<00:20, 8.20it/s]
Rotationally aligning classes: 18%|█▊ | 35/200 [00:03<00:20, 8.17it/s]
Rotationally aligning classes: 18%|█▊ | 36/200 [00:04<00:20, 8.16it/s]
Rotationally aligning classes: 18%|█▊ | 37/200 [00:04<00:19, 8.19it/s]
Rotationally aligning classes: 19%|█▉ | 38/200 [00:04<00:19, 8.20it/s]
Rotationally aligning classes: 20%|█▉ | 39/200 [00:04<00:18, 8.58it/s]
Rotationally aligning classes: 20%|██ | 41/200 [00:04<00:16, 9.66it/s]
Rotationally aligning classes: 21%|██ | 42/200 [00:04<00:16, 9.30it/s]
Rotationally aligning classes: 22%|██▏ | 43/200 [00:04<00:17, 9.03it/s]
Rotationally aligning classes: 22%|██▏ | 44/200 [00:04<00:17, 8.79it/s]
Rotationally aligning classes: 23%|██▎ | 46/200 [00:05<00:15, 9.93it/s]
Rotationally aligning classes: 24%|██▎ | 47/200 [00:05<00:16, 9.50it/s]
Rotationally aligning classes: 24%|██▍ | 48/200 [00:05<00:16, 9.17it/s]
Rotationally aligning classes: 24%|██▍ | 49/200 [00:05<00:17, 8.86it/s]
Rotationally aligning classes: 25%|██▌ | 50/200 [00:05<00:17, 8.70it/s]
Rotationally aligning classes: 26%|██▌ | 51/200 [00:05<00:17, 8.58it/s]
Rotationally aligning classes: 26%|██▋ | 53/200 [00:05<00:16, 8.87it/s]
Rotationally aligning classes: 28%|██▊ | 55/200 [00:06<00:15, 9.41it/s]
Rotationally aligning classes: 28%|██▊ | 56/200 [00:06<00:15, 9.10it/s]
Rotationally aligning classes: 28%|██▊ | 57/200 [00:06<00:16, 8.77it/s]
Rotationally aligning classes: 29%|██▉ | 58/200 [00:06<00:16, 8.62it/s]
Rotationally aligning classes: 30%|██▉ | 59/200 [00:06<00:16, 8.50it/s]
Rotationally aligning classes: 30%|███ | 60/200 [00:06<00:16, 8.42it/s]
Rotationally aligning classes: 30%|███ | 61/200 [00:06<00:16, 8.29it/s]
Rotationally aligning classes: 31%|███ | 62/200 [00:06<00:16, 8.27it/s]
Rotationally aligning classes: 32%|███▏ | 63/200 [00:07<00:16, 8.22it/s]
Rotationally aligning classes: 32%|███▏ | 64/200 [00:07<00:16, 8.29it/s]
Rotationally aligning classes: 32%|███▎ | 65/200 [00:07<00:16, 8.33it/s]
Rotationally aligning classes: 33%|███▎ | 66/200 [00:07<00:16, 8.27it/s]
Rotationally aligning classes: 34%|███▎ | 67/200 [00:07<00:16, 8.27it/s]
Rotationally aligning classes: 34%|███▍ | 68/200 [00:07<00:16, 8.21it/s]
Rotationally aligning classes: 34%|███▍ | 69/200 [00:07<00:15, 8.25it/s]
Rotationally aligning classes: 35%|███▌ | 70/200 [00:07<00:15, 8.24it/s]
Rotationally aligning classes: 36%|███▌ | 71/200 [00:08<00:15, 8.19it/s]
Rotationally aligning classes: 36%|███▌ | 72/200 [00:08<00:15, 8.16it/s]
Rotationally aligning classes: 36%|███▋ | 73/200 [00:08<00:15, 8.21it/s]
Rotationally aligning classes: 37%|███▋ | 74/200 [00:08<00:15, 8.22it/s]
Rotationally aligning classes: 38%|███▊ | 75/200 [00:08<00:14, 8.51it/s]
Rotationally aligning classes: 38%|███▊ | 76/200 [00:08<00:14, 8.41it/s]
Rotationally aligning classes: 38%|███▊ | 77/200 [00:08<00:14, 8.34it/s]
Rotationally aligning classes: 39%|███▉ | 78/200 [00:08<00:14, 8.20it/s]
Rotationally aligning classes: 40%|███▉ | 79/200 [00:09<00:14, 8.35it/s]
Rotationally aligning classes: 40%|████ | 81/200 [00:09<00:11, 9.97it/s]
Rotationally aligning classes: 41%|████ | 82/200 [00:09<00:12, 9.43it/s]
Rotationally aligning classes: 42%|████▏ | 83/200 [00:09<00:13, 8.92it/s]
Rotationally aligning classes: 42%|████▏ | 84/200 [00:09<00:13, 8.70it/s]
Rotationally aligning classes: 42%|████▎ | 85/200 [00:09<00:13, 8.44it/s]
Rotationally aligning classes: 43%|████▎ | 86/200 [00:09<00:13, 8.41it/s]
Rotationally aligning classes: 44%|████▎ | 87/200 [00:09<00:13, 8.35it/s]
Rotationally aligning classes: 44%|████▍ | 88/200 [00:10<00:13, 8.34it/s]
Rotationally aligning classes: 45%|████▌ | 90/200 [00:10<00:10, 10.91it/s]
Rotationally aligning classes: 46%|████▌ | 92/200 [00:10<00:09, 10.94it/s]
Rotationally aligning classes: 47%|████▋ | 94/200 [00:10<00:10, 9.79it/s]
Rotationally aligning classes: 48%|████▊ | 96/200 [00:10<00:09, 10.51it/s]
Rotationally aligning classes: 49%|████▉ | 98/200 [00:11<00:10, 9.69it/s]
Rotationally aligning classes: 50%|█████ | 100/200 [00:11<00:09, 10.14it/s]
Rotationally aligning classes: 51%|█████ | 102/200 [00:11<00:09, 10.61it/s]
Rotationally aligning classes: 52%|█████▏ | 104/200 [00:11<00:08, 10.76it/s]
Rotationally aligning classes: 53%|█████▎ | 106/200 [00:11<00:09, 9.82it/s]
Rotationally aligning classes: 54%|█████▍ | 108/200 [00:11<00:09, 10.10it/s]
Rotationally aligning classes: 55%|█████▌ | 110/200 [00:12<00:09, 9.54it/s]
Rotationally aligning classes: 56%|█████▌ | 112/200 [00:12<00:08, 10.08it/s]
Rotationally aligning classes: 57%|█████▋ | 114/200 [00:12<00:09, 9.55it/s]
Rotationally aligning classes: 57%|█████▊ | 115/200 [00:12<00:09, 9.06it/s]
Rotationally aligning classes: 58%|█████▊ | 116/200 [00:12<00:09, 8.90it/s]
Rotationally aligning classes: 59%|█████▉ | 118/200 [00:13<00:08, 9.94it/s]
Rotationally aligning classes: 60%|█████▉ | 119/200 [00:13<00:08, 9.55it/s]
Rotationally aligning classes: 60%|██████ | 120/200 [00:13<00:08, 9.24it/s]
Rotationally aligning classes: 60%|██████ | 121/200 [00:13<00:08, 8.96it/s]
Rotationally aligning classes: 61%|██████ | 122/200 [00:13<00:08, 8.83it/s]
Rotationally aligning classes: 62%|██████▏ | 123/200 [00:13<00:08, 8.70it/s]
Rotationally aligning classes: 62%|██████▏ | 124/200 [00:13<00:08, 8.53it/s]
Rotationally aligning classes: 62%|██████▎ | 125/200 [00:13<00:08, 8.44it/s]
Rotationally aligning classes: 63%|██████▎ | 126/200 [00:13<00:08, 8.47it/s]
Rotationally aligning classes: 64%|██████▎ | 127/200 [00:14<00:08, 8.80it/s]
Rotationally aligning classes: 64%|██████▍ | 128/200 [00:14<00:08, 8.63it/s]
Rotationally aligning classes: 64%|██████▍ | 129/200 [00:14<00:08, 8.54it/s]
Rotationally aligning classes: 65%|██████▌ | 130/200 [00:14<00:08, 8.46it/s]
Rotationally aligning classes: 66%|██████▌ | 132/200 [00:14<00:07, 9.65it/s]
Rotationally aligning classes: 66%|██████▋ | 133/200 [00:14<00:07, 9.25it/s]
Rotationally aligning classes: 67%|██████▋ | 134/200 [00:14<00:07, 8.92it/s]
Rotationally aligning classes: 68%|██████▊ | 135/200 [00:14<00:07, 8.69it/s]
Rotationally aligning classes: 68%|██████▊ | 136/200 [00:15<00:07, 8.57it/s]
Rotationally aligning classes: 68%|██████▊ | 137/200 [00:15<00:07, 8.34it/s]
Rotationally aligning classes: 69%|██████▉ | 138/200 [00:15<00:07, 8.26it/s]
Rotationally aligning classes: 70%|██████▉ | 139/200 [00:15<00:07, 8.20it/s]
Rotationally aligning classes: 70%|███████ | 140/200 [00:15<00:07, 8.17it/s]
Rotationally aligning classes: 70%|███████ | 141/200 [00:15<00:07, 8.21it/s]
Rotationally aligning classes: 72%|███████▏ | 143/200 [00:15<00:06, 9.39it/s]
Rotationally aligning classes: 72%|███████▏ | 144/200 [00:16<00:06, 9.10it/s]
Rotationally aligning classes: 72%|███████▎ | 145/200 [00:16<00:06, 8.83it/s]
Rotationally aligning classes: 73%|███████▎ | 146/200 [00:16<00:06, 8.64it/s]
Rotationally aligning classes: 74%|███████▎ | 147/200 [00:16<00:06, 8.52it/s]
Rotationally aligning classes: 74%|███████▍ | 148/200 [00:16<00:06, 8.47it/s]
Rotationally aligning classes: 74%|███████▍ | 149/200 [00:16<00:06, 8.43it/s]
Rotationally aligning classes: 75%|███████▌ | 150/200 [00:16<00:05, 8.36it/s]
Rotationally aligning classes: 76%|███████▌ | 151/200 [00:16<00:05, 8.31it/s]
Rotationally aligning classes: 76%|███████▌ | 152/200 [00:17<00:05, 8.26it/s]
Rotationally aligning classes: 76%|███████▋ | 153/200 [00:17<00:05, 8.29it/s]
Rotationally aligning classes: 77%|███████▋ | 154/200 [00:17<00:05, 8.29it/s]
Rotationally aligning classes: 78%|███████▊ | 155/200 [00:17<00:05, 8.29it/s]
Rotationally aligning classes: 78%|███████▊ | 156/200 [00:17<00:05, 8.20it/s]
Rotationally aligning classes: 78%|███████▊ | 157/200 [00:17<00:05, 8.17it/s]
Rotationally aligning classes: 79%|███████▉ | 158/200 [00:17<00:05, 8.23it/s]
Rotationally aligning classes: 80%|███████▉ | 159/200 [00:17<00:04, 8.26it/s]
Rotationally aligning classes: 80%|████████ | 160/200 [00:17<00:04, 8.31it/s]
Rotationally aligning classes: 81%|████████ | 162/200 [00:18<00:04, 9.46it/s]
Rotationally aligning classes: 82%|████████▏ | 163/200 [00:18<00:04, 9.08it/s]
Rotationally aligning classes: 82%|████████▏ | 164/200 [00:18<00:04, 8.81it/s]
Rotationally aligning classes: 82%|████████▎ | 165/200 [00:18<00:04, 8.63it/s]
Rotationally aligning classes: 83%|████████▎ | 166/200 [00:18<00:03, 8.57it/s]
Rotationally aligning classes: 84%|████████▎ | 167/200 [00:18<00:03, 8.44it/s]
Rotationally aligning classes: 84%|████████▍ | 168/200 [00:18<00:03, 8.36it/s]
Rotationally aligning classes: 84%|████████▍ | 169/200 [00:19<00:03, 8.32it/s]
Rotationally aligning classes: 85%|████████▌ | 170/200 [00:19<00:03, 8.40it/s]
Rotationally aligning classes: 86%|████████▌ | 172/200 [00:19<00:03, 8.83it/s]
Rotationally aligning classes: 86%|████████▋ | 173/200 [00:19<00:03, 8.63it/s]
Rotationally aligning classes: 87%|████████▋ | 174/200 [00:19<00:03, 8.55it/s]
Rotationally aligning classes: 88%|████████▊ | 175/200 [00:19<00:02, 8.45it/s]
Rotationally aligning classes: 88%|████████▊ | 176/200 [00:19<00:02, 8.76it/s]
Rotationally aligning classes: 89%|████████▉ | 178/200 [00:20<00:02, 9.04it/s]
Rotationally aligning classes: 90%|████████▉ | 179/200 [00:20<00:02, 8.73it/s]
Rotationally aligning classes: 90%|█████████ | 180/200 [00:20<00:02, 8.62it/s]
Rotationally aligning classes: 90%|█████████ | 181/200 [00:20<00:02, 8.54it/s]
Rotationally aligning classes: 91%|█████████ | 182/200 [00:20<00:02, 8.45it/s]
Rotationally aligning classes: 92%|█████████▏| 183/200 [00:20<00:02, 8.39it/s]
Rotationally aligning classes: 92%|█████████▏| 184/200 [00:20<00:01, 8.51it/s]
Rotationally aligning classes: 93%|█████████▎| 186/200 [00:20<00:01, 8.82it/s]
Rotationally aligning classes: 94%|█████████▎| 187/200 [00:21<00:01, 8.65it/s]
Rotationally aligning classes: 94%|█████████▍| 188/200 [00:21<00:01, 8.53it/s]
Rotationally aligning classes: 94%|█████████▍| 189/200 [00:21<00:01, 8.53it/s]
Rotationally aligning classes: 95%|█████████▌| 190/200 [00:21<00:01, 8.41it/s]
Rotationally aligning classes: 96%|█████████▌| 191/200 [00:21<00:01, 8.38it/s]
Rotationally aligning classes: 96%|█████████▌| 192/200 [00:21<00:00, 8.33it/s]
Rotationally aligning classes: 96%|█████████▋| 193/200 [00:21<00:00, 8.58it/s]
Rotationally aligning classes: 97%|█████████▋| 194/200 [00:21<00:00, 8.53it/s]
Rotationally aligning classes: 98%|█████████▊| 195/200 [00:22<00:00, 8.46it/s]
Rotationally aligning classes: 98%|█████████▊| 196/200 [00:22<00:00, 8.42it/s]
Rotationally aligning classes: 98%|█████████▊| 197/200 [00:22<00:00, 8.39it/s]
Rotationally aligning classes: 99%|█████████▉| 198/200 [00:22<00:00, 8.65it/s]
Rotationally aligning classes: 100%|█████████▉| 199/200 [00:22<00:00, 8.61it/s]
Rotationally aligning classes: 100%|██████████| 200/200 [00:22<00:00, 8.62it/s]
Rotationally aligning classes: 100%|██████████| 200/200 [00:22<00:00, 8.84it/s]
Stacking and evaluating class averages from FFBBasis2D to Cartesian: 0%| | 0/1 [00:00<?, ?it/s]
Stacking batch: 0%| | 0/200 [00:00<?, ?it/s]
Stacking batch: 1%| | 2/200 [00:00<00:10, 19.42it/s]
Stacking batch: 12%|█▏ | 24/200 [00:00<00:01, 134.66it/s]
Stacking batch: 24%|██▎ | 47/200 [00:00<00:00, 174.83it/s]
Stacking batch: 35%|███▌ | 70/200 [00:00<00:00, 193.82it/s]
Stacking batch: 46%|████▋ | 93/200 [00:00<00:00, 204.17it/s]
Stacking batch: 58%|█████▊ | 116/200 [00:00<00:00, 210.26it/s]
Stacking batch: 70%|██████▉ | 139/200 [00:00<00:00, 214.50it/s]
Stacking batch: 80%|████████ | 161/200 [00:00<00:00, 213.18it/s]
Stacking batch: 92%|█████████▏| 184/200 [00:00<00:00, 216.36it/s]
Stacking and evaluating class averages from FFBBasis2D to Cartesian: 100%|██████████| 1/1 [00:01<00:00, 1.10s/it]
Stacking and evaluating class averages from FFBBasis2D to Cartesian: 100%|██████████| 1/1 [00:01<00:00, 1.10s/it]
100%|██████████| 1/1 [00:28<00:00, 28.13s/it]
100%|██████████| 1/1 [00:28<00:00, 28.13s/it]
View the Class Averages¶
# Show class averages
avgs.images[0:10].show()
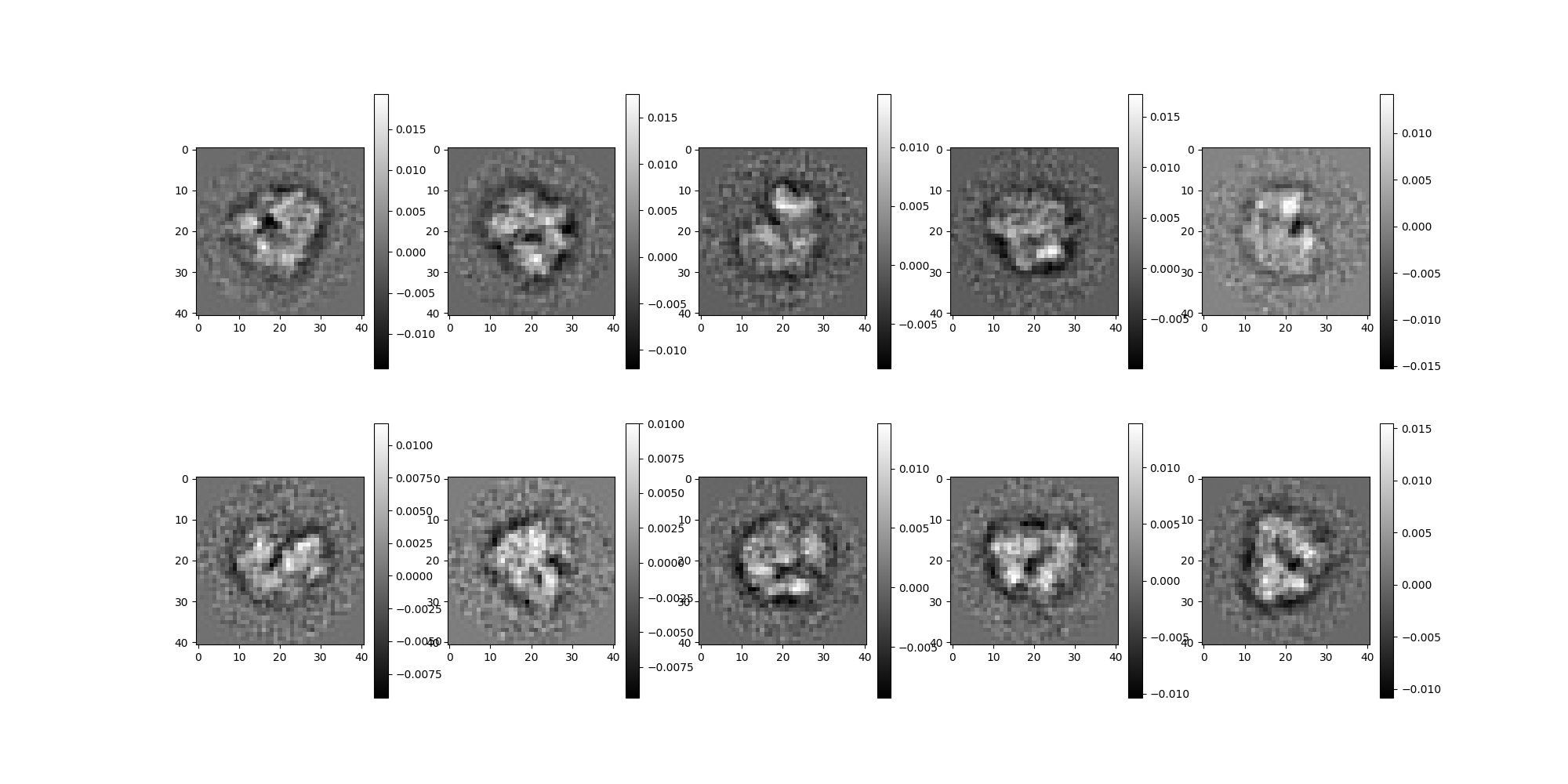
# Show original images corresponding to those classes. This 1:1
# comparison is only expected to work because we used
# ``TopClassSelector`` to classify our images.
src.images[0:10].show()
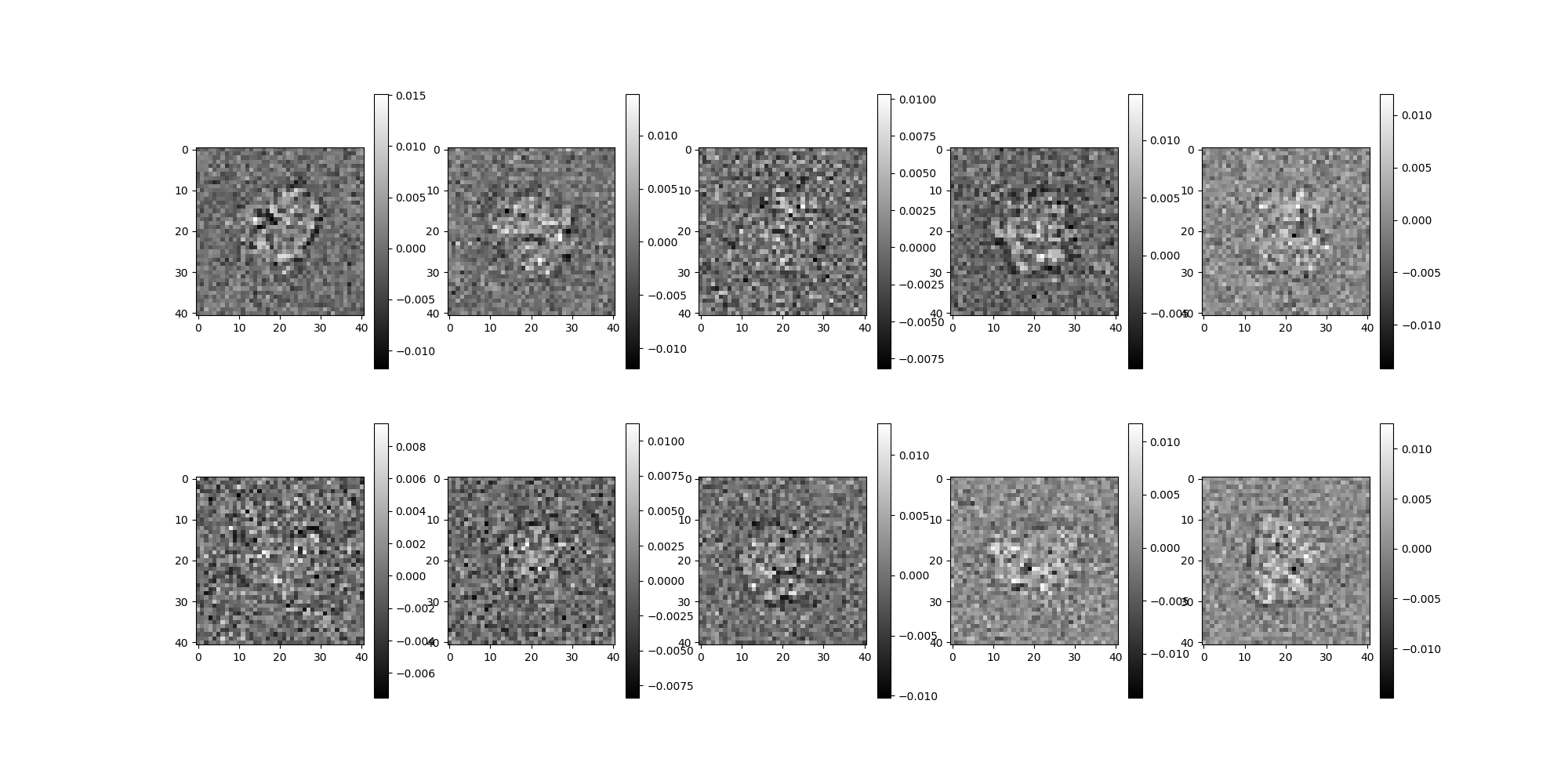
Orientation Estimation¶
We create an OrientedSource
, which consumes an ImageSource
object, an
orientation estimator, and returns a new source which lazily estimates orientations.
In this case we supply avgs
for our source and a CLSyncVoting
class instance for our orientation estimator. The CLSyncVoting
algorithm employs
a common-lines method with synchronization and voting.
from aspire.abinitio import CLSyncVoting
from aspire.source import OrientedSource
# Stash true rotations for later comparison
true_rotations = src.rotations[:n_classes]
# For this low resolution example we will customize the ``CLSyncVoting``
# instance to use fewer theta points ``n_theta`` then the default value of 360.
orient_est = CLSyncVoting(avgs, n_theta=72)
# Instantiate an ``OrientedSource``.
oriented_src = OrientedSource(avgs, orient_est)
Mean Error of Estimated Rotations¶
ASPIRE has the built-in utility function, mean_aligned_angular_distance
, which globally
aligns the estimated rotations to the true rotations and computes the mean
angular distance (in degrees).
from aspire.utils import mean_aligned_angular_distance
# Compare with known true rotations
mean_ang_dist = mean_aligned_angular_distance(oriented_src.rotations, true_rotations)
print(f"Mean aligned angular distance: {mean_ang_dist} degrees")
Searching over common line pairs: 0%| | 0/19900 [00:00<?, ?it/s]
Searching over common line pairs: 2%|▏ | 314/19900 [00:00<00:06, 3136.26it/s]
Searching over common line pairs: 3%|▎ | 636/19900 [00:00<00:06, 3184.17it/s]
Searching over common line pairs: 5%|▍ | 959/19900 [00:00<00:05, 3202.36it/s]
Searching over common line pairs: 6%|▋ | 1281/19900 [00:00<00:05, 3209.13it/s]
Searching over common line pairs: 8%|▊ | 1602/19900 [00:00<00:05, 3203.55it/s]
Searching over common line pairs: 10%|▉ | 1926/19900 [00:00<00:05, 3213.26it/s]
Searching over common line pairs: 11%|█▏ | 2248/19900 [00:00<00:05, 3214.59it/s]
Searching over common line pairs: 13%|█▎ | 2570/19900 [00:00<00:05, 3209.58it/s]
Searching over common line pairs: 15%|█▍ | 2893/19900 [00:00<00:05, 3213.31it/s]
Searching over common line pairs: 16%|█▌ | 3218/19900 [00:01<00:05, 3221.94it/s]
Searching over common line pairs: 18%|█▊ | 3541/19900 [00:01<00:05, 3215.69it/s]
Searching over common line pairs: 19%|█▉ | 3863/19900 [00:01<00:04, 3209.87it/s]
Searching over common line pairs: 21%|██ | 4184/19900 [00:01<00:04, 3199.68it/s]
Searching over common line pairs: 23%|██▎ | 4507/19900 [00:01<00:04, 3207.08it/s]
Searching over common line pairs: 24%|██▍ | 4829/19900 [00:01<00:04, 3209.07it/s]
Searching over common line pairs: 26%|██▌ | 5152/19900 [00:01<00:04, 3214.47it/s]
Searching over common line pairs: 28%|██▊ | 5474/19900 [00:01<00:04, 3215.90it/s]
Searching over common line pairs: 29%|██▉ | 5796/19900 [00:01<00:04, 3216.38it/s]
Searching over common line pairs: 31%|███ | 6118/19900 [00:01<00:04, 3208.48it/s]
Searching over common line pairs: 32%|███▏ | 6440/19900 [00:02<00:04, 3209.06it/s]
Searching over common line pairs: 34%|███▍ | 6761/19900 [00:02<00:04, 3208.29it/s]
Searching over common line pairs: 36%|███▌ | 7082/19900 [00:02<00:04, 3203.40it/s]
Searching over common line pairs: 37%|███▋ | 7403/19900 [00:02<00:03, 3198.89it/s]
Searching over common line pairs: 39%|███▉ | 7724/19900 [00:02<00:03, 3201.69it/s]
Searching over common line pairs: 40%|████ | 8045/19900 [00:02<00:03, 3196.83it/s]
Searching over common line pairs: 42%|████▏ | 8367/19900 [00:02<00:03, 3203.31it/s]
Searching over common line pairs: 44%|████▎ | 8689/19900 [00:02<00:03, 3206.84it/s]
Searching over common line pairs: 45%|████▌ | 9011/19900 [00:02<00:03, 3210.68it/s]
Searching over common line pairs: 47%|████▋ | 9333/19900 [00:02<00:03, 3204.55it/s]
Searching over common line pairs: 49%|████▊ | 9654/19900 [00:03<00:03, 3202.44it/s]
Searching over common line pairs: 50%|█████ | 9975/19900 [00:03<00:03, 3203.22it/s]
Searching over common line pairs: 52%|█████▏ | 10298/19900 [00:03<00:02, 3210.32it/s]
Searching over common line pairs: 53%|█████▎ | 10620/19900 [00:03<00:02, 3212.88it/s]
Searching over common line pairs: 55%|█████▍ | 10942/19900 [00:03<00:02, 3213.44it/s]
Searching over common line pairs: 57%|█████▋ | 11264/19900 [00:03<00:02, 3197.69it/s]
Searching over common line pairs: 58%|█████▊ | 11588/19900 [00:03<00:02, 3207.66it/s]
Searching over common line pairs: 60%|█████▉ | 11911/19900 [00:03<00:02, 3213.02it/s]
Searching over common line pairs: 61%|██████▏ | 12234/19900 [00:03<00:02, 3216.02it/s]
Searching over common line pairs: 63%|██████▎ | 12557/19900 [00:03<00:02, 3219.02it/s]
Searching over common line pairs: 65%|██████▍ | 12879/19900 [00:04<00:02, 3217.67it/s]
Searching over common line pairs: 66%|██████▋ | 13201/19900 [00:04<00:02, 3215.18it/s]
Searching over common line pairs: 68%|██████▊ | 13523/19900 [00:04<00:01, 3216.37it/s]
Searching over common line pairs: 70%|██████▉ | 13845/19900 [00:04<00:01, 3216.52it/s]
Searching over common line pairs: 71%|███████ | 14168/19900 [00:04<00:01, 3218.07it/s]
Searching over common line pairs: 73%|███████▎ | 14490/19900 [00:04<00:01, 3217.65it/s]
Searching over common line pairs: 74%|███████▍ | 14812/19900 [00:04<00:01, 3215.88it/s]
Searching over common line pairs: 76%|███████▌ | 15135/19900 [00:04<00:01, 3218.01it/s]
Searching over common line pairs: 78%|███████▊ | 15458/19900 [00:04<00:01, 3220.05it/s]
Searching over common line pairs: 79%|███████▉ | 15781/19900 [00:04<00:01, 3219.98it/s]
Searching over common line pairs: 81%|████████ | 16103/19900 [00:05<00:01, 3212.68it/s]
Searching over common line pairs: 83%|████████▎ | 16425/19900 [00:05<00:01, 3214.56it/s]
Searching over common line pairs: 84%|████████▍ | 16748/19900 [00:05<00:00, 3217.62it/s]
Searching over common line pairs: 86%|████████▌ | 17070/19900 [00:05<00:00, 3211.77it/s]
Searching over common line pairs: 87%|████████▋ | 17392/19900 [00:05<00:00, 3213.47it/s]
Searching over common line pairs: 89%|████████▉ | 17715/19900 [00:05<00:00, 3215.68it/s]
Searching over common line pairs: 91%|█████████ | 18039/19900 [00:05<00:00, 3222.36it/s]
Searching over common line pairs: 92%|█████████▏| 18363/19900 [00:05<00:00, 3225.36it/s]
Searching over common line pairs: 94%|█████████▍| 18686/19900 [00:05<00:00, 3220.32it/s]
Searching over common line pairs: 96%|█████████▌| 19009/19900 [00:05<00:00, 3216.19it/s]
Searching over common line pairs: 97%|█████████▋| 19332/19900 [00:06<00:00, 3218.47it/s]
Searching over common line pairs: 99%|█████████▉| 19654/19900 [00:06<00:00, 3217.06it/s]
Searching over common line pairs: 100%|██████████| 19900/19900 [00:06<00:00, 3211.17it/s]
LSQR Least-squares solution of Ax = b
The matrix A has 19900 rows and 400 columns
damp = 0.00000000000000e+00 calc_var = 0
atol = 1.00e-06 conlim = 1.00e+08
btol = 1.00e-06 iter_lim = 800
Itn x[0] r1norm r2norm Compatible LS Norm A Cond A
0 0.00000e+00 7.900e+01 7.900e+01 1.0e+00 4.1e-02
1 -9.09180e-02 7.372e+01 7.372e+01 9.3e-01 7.6e-02 9.1e+00 1.0e+00
2 -6.20053e-02 7.347e+01 7.347e+01 9.3e-01 1.5e-02 1.3e+01 2.0e+00
3 -7.09242e-02 7.345e+01 7.345e+01 9.3e-01 4.6e-03 1.5e+01 3.1e+00
4 -6.65593e-02 7.345e+01 7.345e+01 9.3e-01 2.2e-03 1.7e+01 4.3e+00
5 -6.65370e-02 7.345e+01 7.345e+01 9.3e-01 9.2e-04 1.9e+01 5.7e+00
6 -6.57091e-02 7.345e+01 7.345e+01 9.3e-01 3.0e-04 2.1e+01 6.9e+00
7 -6.61623e-02 7.345e+01 7.345e+01 9.3e-01 1.1e-04 2.2e+01 8.1e+00
8 -6.59082e-02 7.345e+01 7.345e+01 9.3e-01 4.6e-05 2.3e+01 9.3e+00
9 -6.60291e-02 7.345e+01 7.345e+01 9.3e-01 1.9e-05 2.5e+01 1.0e+01
10 -6.59594e-02 7.345e+01 7.345e+01 9.3e-01 7.7e-06 2.6e+01 1.2e+01
11 -6.59790e-02 7.345e+01 7.345e+01 9.3e-01 3.5e-06 2.7e+01 1.3e+01
12 -6.59682e-02 7.345e+01 7.345e+01 9.3e-01 1.3e-06 2.8e+01 1.4e+01
13 -6.59708e-02 7.345e+01 7.345e+01 9.3e-01 4.4e-07 3.0e+01 1.5e+01
LSQR finished
The least-squares solution is good enough, given atol
istop = 2 r1norm = 7.3e+01 anorm = 3.0e+01 arnorm = 9.6e-04
itn = 13 r2norm = 7.3e+01 acond = 1.5e+01 xnorm = 3.4e+00
Mean aligned angular distance: 13.057275772094727 degrees
Volume Reconstruction¶
Now that we have our class averages and rotation estimates, we can estimate the mean volume by supplying the class averages and basis for back projection.
from aspire.reconstruction import MeanEstimator
# Setup an estimator to perform the back projection.
estimator = MeanEstimator(oriented_src)
# Perform the estimation and save the volume.
estimated_volume = estimator.estimate()
Comparison of Estimated Volume with Source Volume¶
To get a visual confirmation that our results are sane, we rotate the estimated volume by the estimated rotations and project along the z-axis. These estimated projections should align with the original projection images.
# Get the first 10 projections from the estimated volume using the
# estimated orientations. Recall that ``project`` returns an
# ``Image`` instance, which we can peek at with ``show``.
projections_est = estimated_volume.project(oriented_src.rotations[0:10])
# We view the first 10 projections of the estimated volume.
projections_est.show()
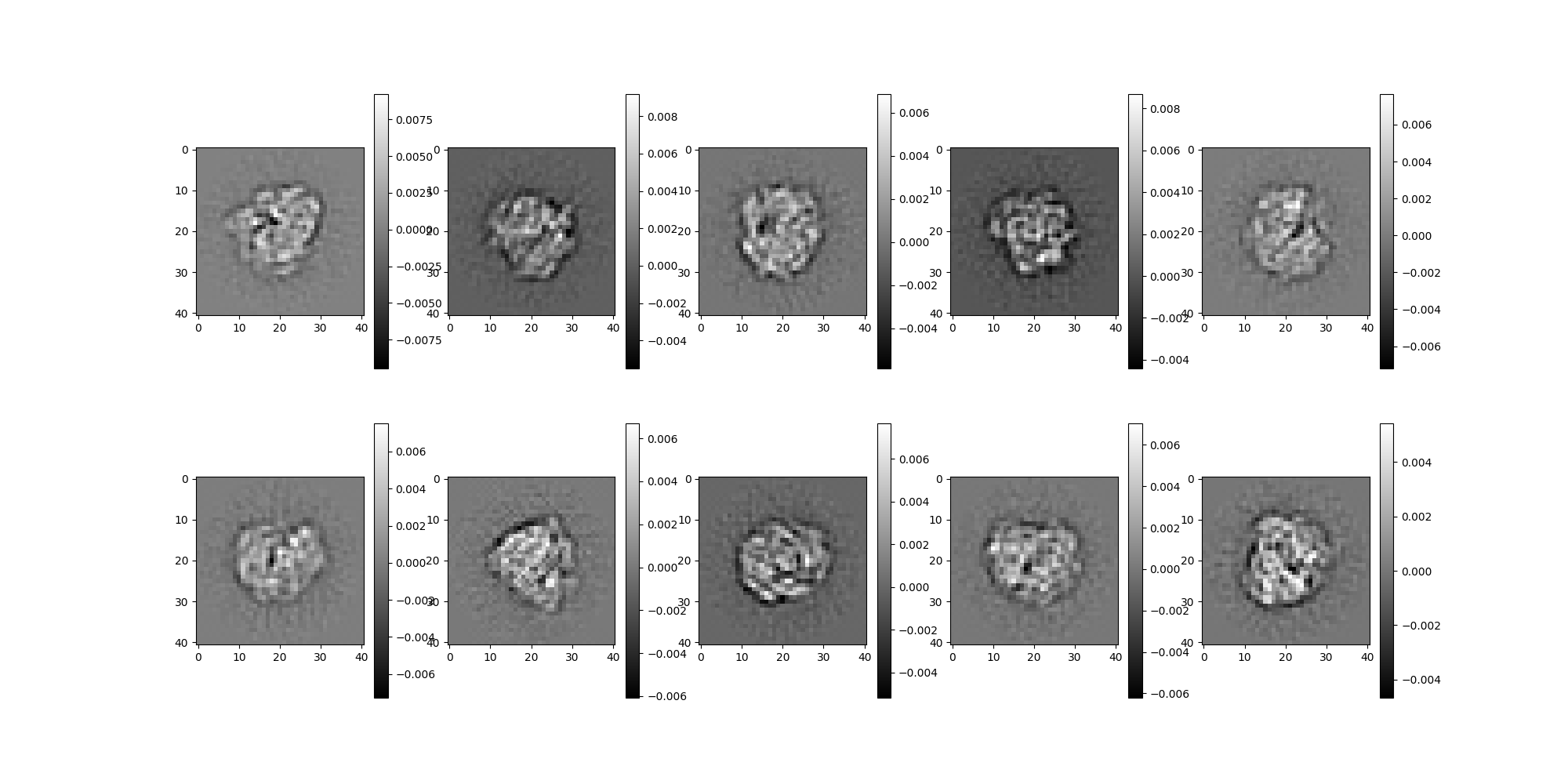
# For comparison, we view the first 10 source projections.
src.projections[0:10].show()
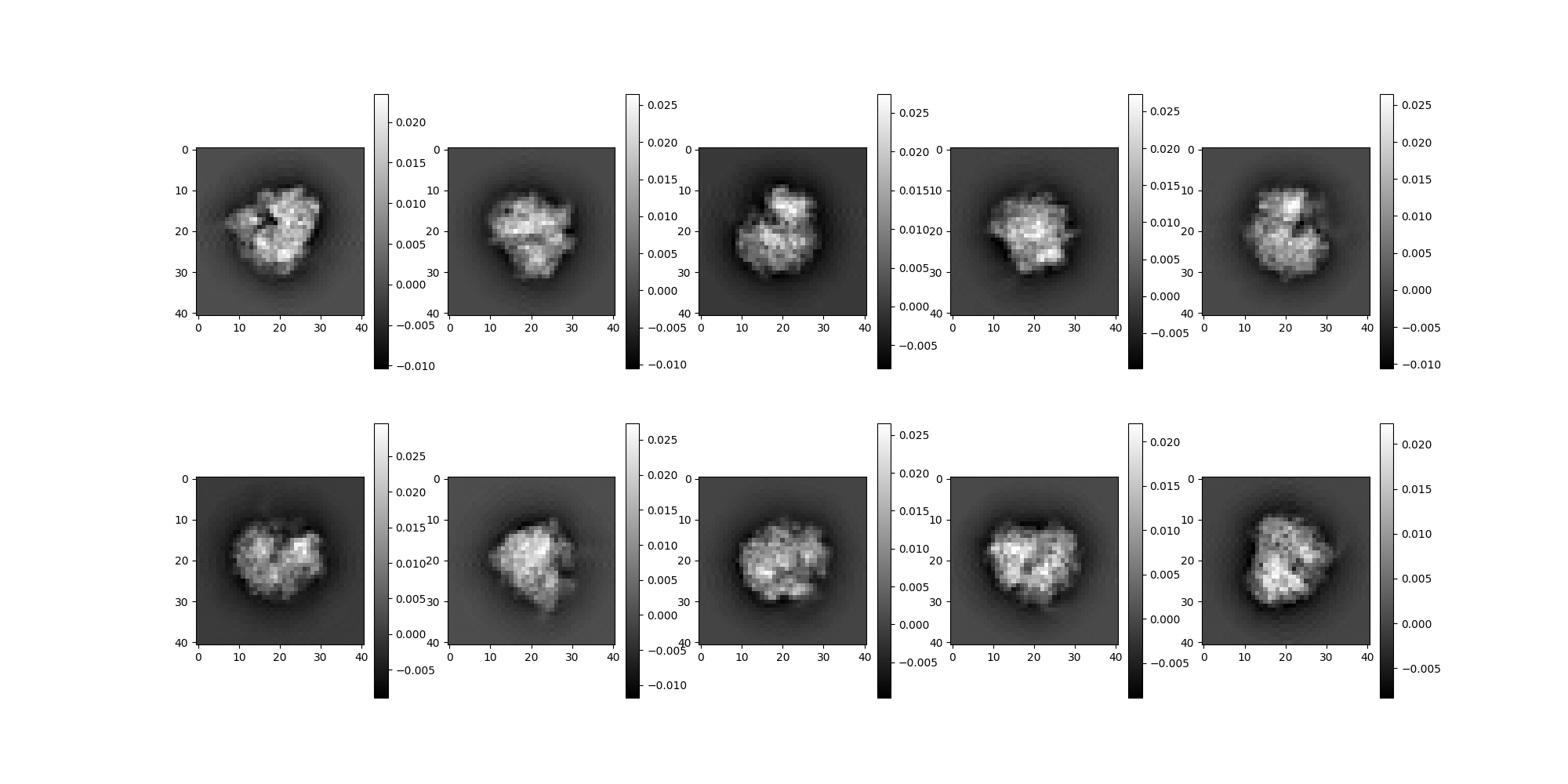
Total running time of the script: (1 minutes 6.530 seconds)